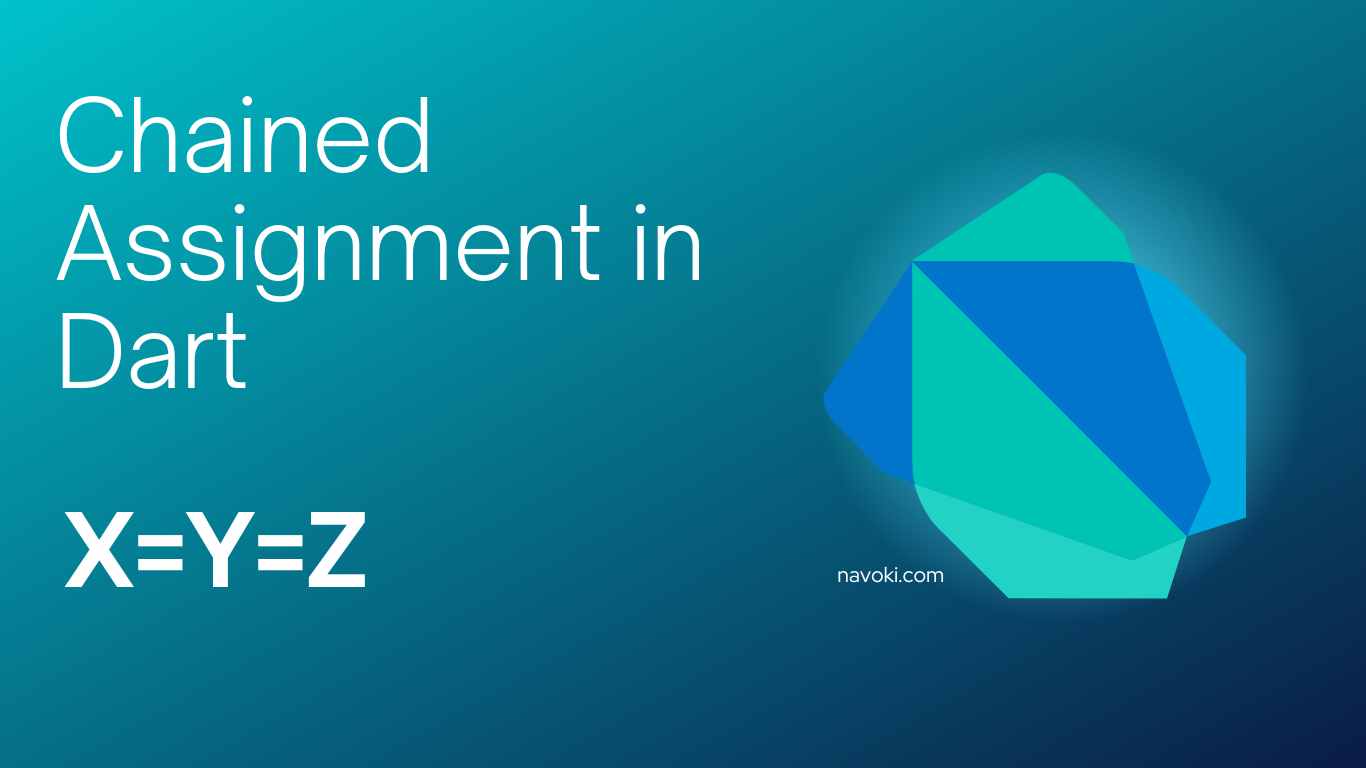
The Chain Assignment Trick : Dart Programming
Want to write cleaner, more efficient code for your Flutter mobile apps? Dart programming language behind Flutter, has a handy feature called chain assignment that can help you do just that.
What is Chain Assignment?
Picture this: You’re baking cookies and need to set multiple timers. Instead of setting each one individually, wouldn’t it be great to set them all at once? That’s the idea behind chain assignment.
In Dart, you can assign the same value to multiple variables using a single line of code:
void main() { int x=10; int y=5; int z=2; x=y=z; print("x=$x, y=$y, z=$z"); }
In this example, we first create three variables: x
, y
, and z
. Then, we use chain assignment to set all three variables equal to the value of z
, which is 2.
How Does It Work?
Chain assignment works like a domino effect, starting from the rightmost variable and moving left. The value of z
is assigned to y
, and then the value of y
(now 2) is assigned to x
.
Why Use Chain Assignment in Flutter Mobile App Development?
- Concise Code: It makes your code shorter and easier to read, especially when you’re dealing with many variables.
- Improved Efficiency: In some cases, it can be slightly faster than assigning values one by one.
- Consistent Values: It ensures that all variables in the chain have the same value.
Things to Keep in Mind
- Chain assignment works best when you want to assign the same value to multiple variables.
- Use it thoughtfully. In complex scenarios, excessive chain assignments might make your code harder to understand.
If you’re looking to master Dart programming and build awesome Flutter mobile apps, chain assignment is a simple but effective technique to add to your toolkit. Happy coding!