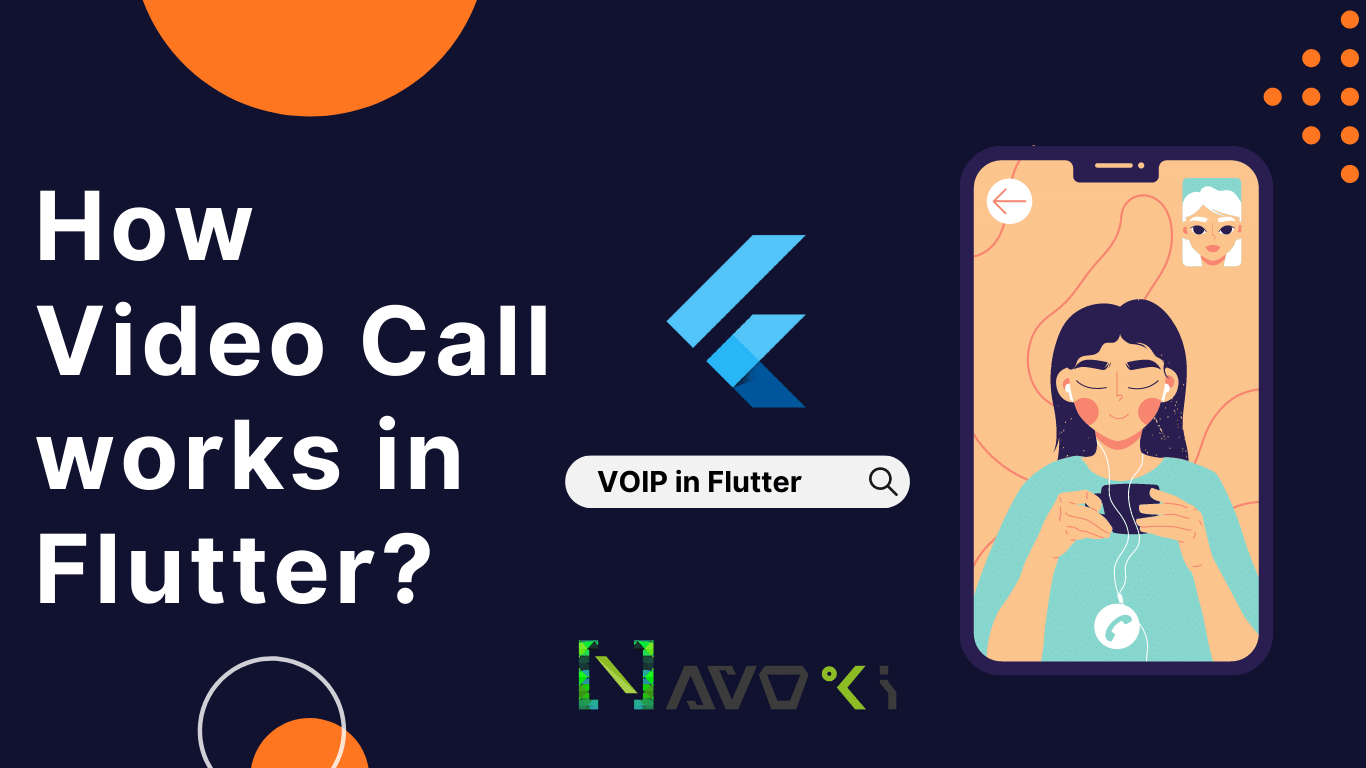
How Incoming Video Call Notification works in Flutter
Introduction
How What’s App call works and How to implement flutter video call. It is a pretty complicated and tedious task for a junior developer to implement it for the first time. Let us see how well this blog will explain the implementation of Voice-over-Internet Protocol (VoIP) in Android and iOS using Flutter. The basic flow of flutter VoIP push notification is shown in the image below:
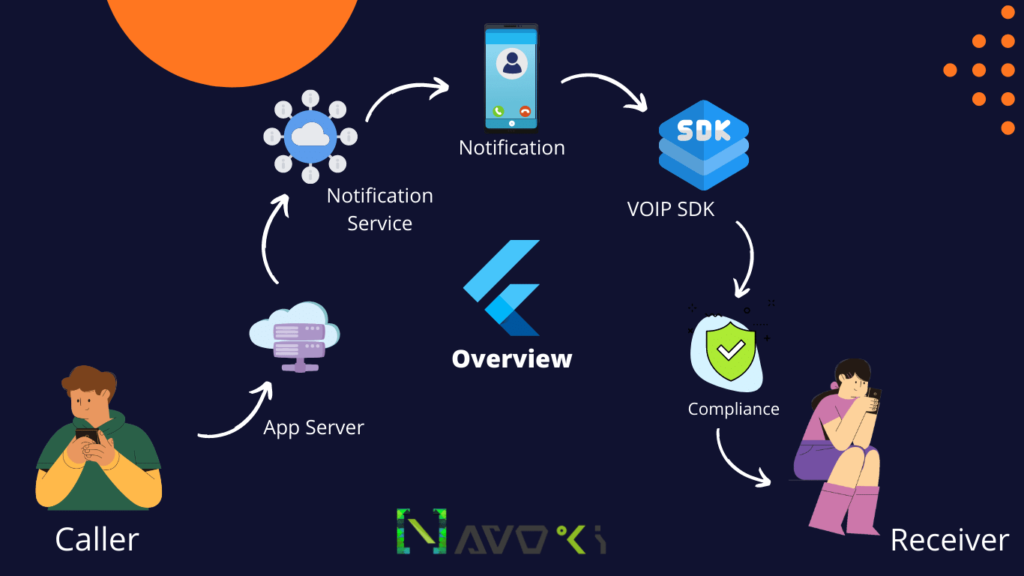
VoIP Services in Flutter app can be divided into 2 parts:
- Implement SDK to add VoIP feature in app. Any one of below can be used
- WEBRTC in Flutter, to add real-time communication capabilities to your application.
- AGORA Flutter SDK, allow you to simply integrate Agora Voice and Video Calling.
- Push Notification for app user for Accept or Reject call.
- Firebase Cloud messaging, Send notification to Android and iOS
- Apple Push Notification service, Send notification to iOS
We need APNs for iOS when Firebase can do the same thing. Firebase cannot be used for iOS for video and voice call services. That will be explained below
VoIP App usage scenario
Sam (User A) is far away from Emma (User B). They need an app where Sam can video calls Emma anytime. Both users have different mobile platforms, Sam has an Android phone, and Emma has iOS. How can we make an app to support flutter video call across platforms?
Video call in Android using Flutter
Create an app for video call in flutter for android; these essential SDK and accounts are required.
- Agora account and SDK is required to provide video and voice call service in flutter.
- Firebase account required to send Cloud Messaging to Android phone in form of push notification.
- Own Server, to handle notification, REST APIs and unique communication channel logic.
- Flutter Project with Android app configured with Firebase. Create a new flutter project by following this tutorial.
The basic flow of Flutter video call in Android is explained and given below.
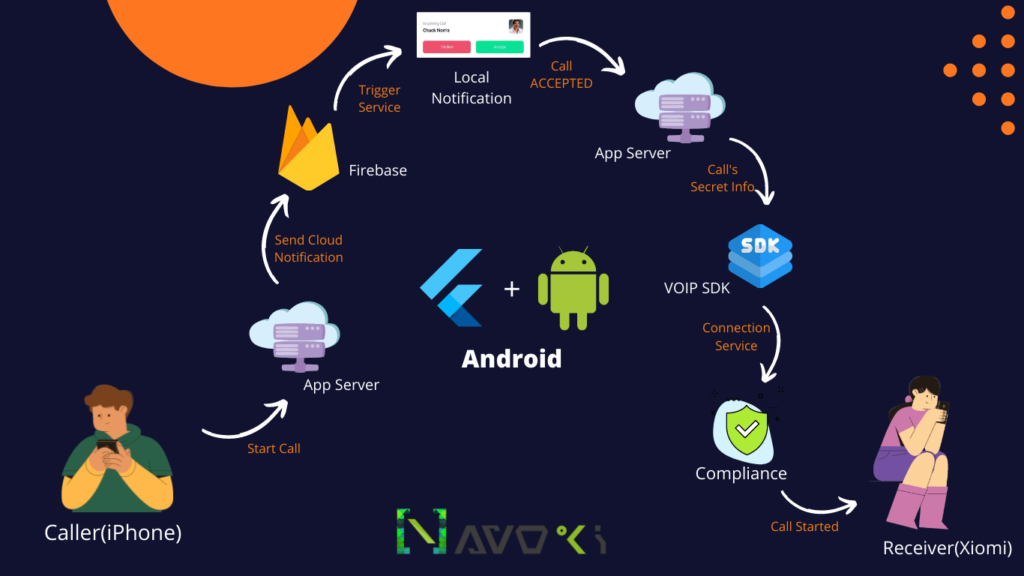
- User B calls to User A, this trigger a Rest API to Server and informed a call is placed.
- The server validates the above request and retrieves the Firebase Device Token of User B. Using this token server, send cloud messaging to User A in the background on an Android device. Payload data for video call notification should be as mentioned below.
data: { session_id: "abc1234567890", // Server record id about communication b/w users caller_name: "User A", // Any String to show Caller's name caller_id: "123456", // Callers id or phone number type: "VOICE_CALL", // Type of call to handle in dart code click_action: "FLUTTER_NOTIFICATION_CLICK", // to listen click on notification in flutter }, android:{ restricted_package_name : "com.navoki.voip", // app package name }
3. It is necessary to send a notification in the above format so that the app can receive it in the background by the app service, and payload data should be processed by our code running in that service.
4. Show incoming call notification to User A to Accept or Reject the call. Custom notification with the button is not supported in flutter_local_notifications code, so an Android native code notification messaging is required. A new package can be used connectycube_flutter_call_kit for Android.
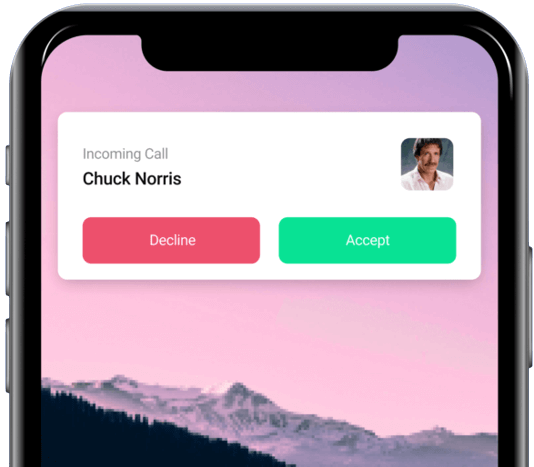
5. If User A was already on another call, ConnectionService will inform in code about this and app should not proceed further to next step, by ending the User A call.
6. Using ConnectionService is optional in Android, so developer can skip if app requirement demands but for best practices implement it.
6. If call ACCEPTED, app call ConnectionService class in Android API to inform the Android device that User A is on call. So if another call comes , VoIP or network call from someone else, they will be informed as “User A on another call” by Android device.
7. Flutter app is launched, and the flutter app receives notification payload data. This information is sent to Server w.r.t session_id
of the call. The server responds with a private channel_id
of Agora SDK used in the app to connect both end-users.
8. Ask Camera and Audio permission from user.
8. Use this channel_id
to connect as voice or video call and shown in form of UI.
Video call in iOS using Flutter
To create a app for video call in flutter for iOS, these essentials SDK and accounts are required.
- Agora account and SDK is required to provide video and voice call service.
- Apple account and APNs certificate required to send messaging to iOS phone in form of VoIP notification.
- Own Server, to handle notification, REST APIs and unique communication channel logic.
- Flutter Project with iOS app configured with Firebase. Create a new flutter project by following this tutorial.
The basic flow of Flutter video call in iOS is explained and given below.
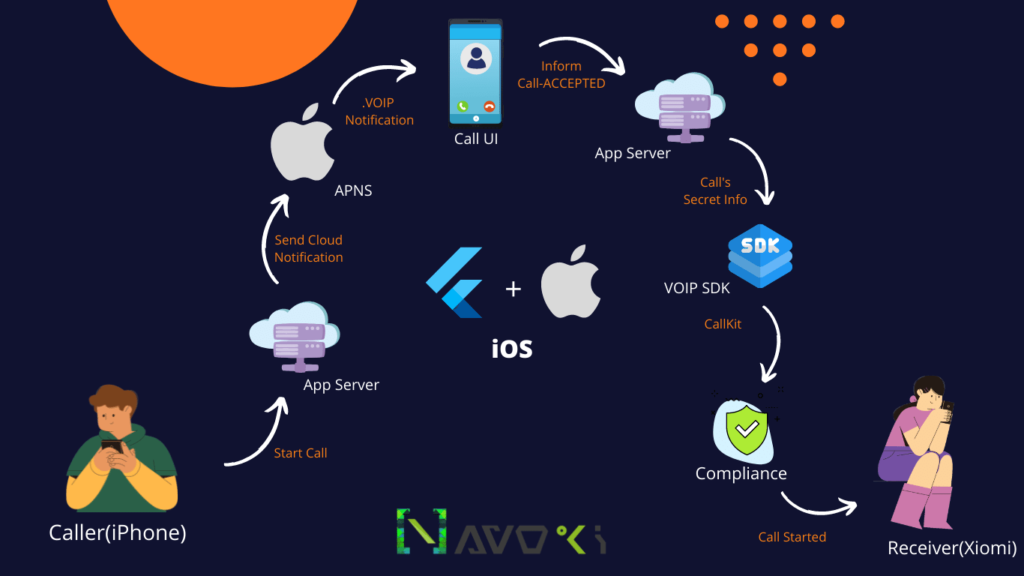
- User A calls to User B, this trigger a Rest API to Server and informed a call is placed.
- Server validates above request and retrieve the iPhone’s UUID of User B.
- Using UUID, a special VoIP push notification is sent using Apple Push Notification. This special message doesn’t invoke any notification instead gives a small window of time to run a code in background. Payload data for video call notification should be as mentioned below
"aps": { "alert": { "uuid": "123", // Receiver's UUID "incoming_caller_id": "123456", // Callers id or phone number "incoming_caller_name": "User A", // Any String to show Caller's name "session_id": "abc1234567890", // Server record id about communication b/w users "type": "VIDEO_CALL", // type of call to handle in dart code "click_action": "FLUTTER_NOTIFICATION_CLICK", }, 'content-available': 1 // IMPORTANT !!! }
4. In iOS, it is mandatory to use CallKit, if app uses flutter VoIP push notification. CallKit code invokes to inform iOS that User is on a ongoing call, almost similar to Android’s ConnectionService.
5. Show iOS native calling UI to Accept or Reject call.
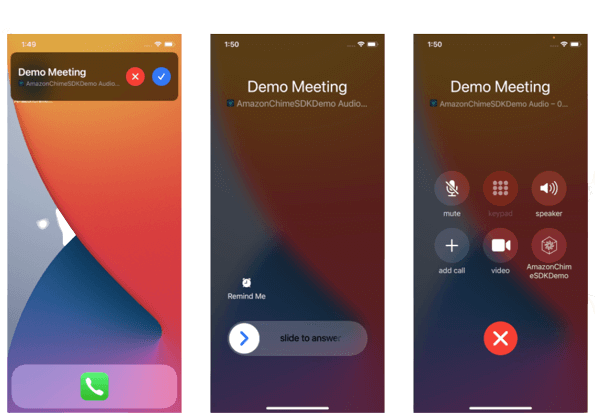
6. If call ACCEPTED, app call CallKit class in iOS informs the iOS device that User B is on call. So if another call comes , voip or network call from someone else they, will be informed as “User A on another call” by iOS device.
7. Flutter app is launched, and the flutter app receives notification payload data. This information is sent to Server w.r.t session_id
of the call. The server responds with a private channel_id
of VoIP SDK used in the app to connect both end-users.
8. Ask Camera and Audio permission from user.
8. Use this channel_id
to connect as voice or video call and shown in form of UI.
Call Connected Successfully !
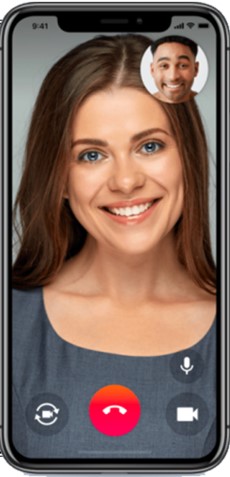
Best Flutter plugins for Video call in Flutter
There are many packages available in pub.dev website. I can suggest these 2 packages which worked for me.
Stuck in Flutter Code? Ask you queries to Flutter Mentor on our Discord Channel.