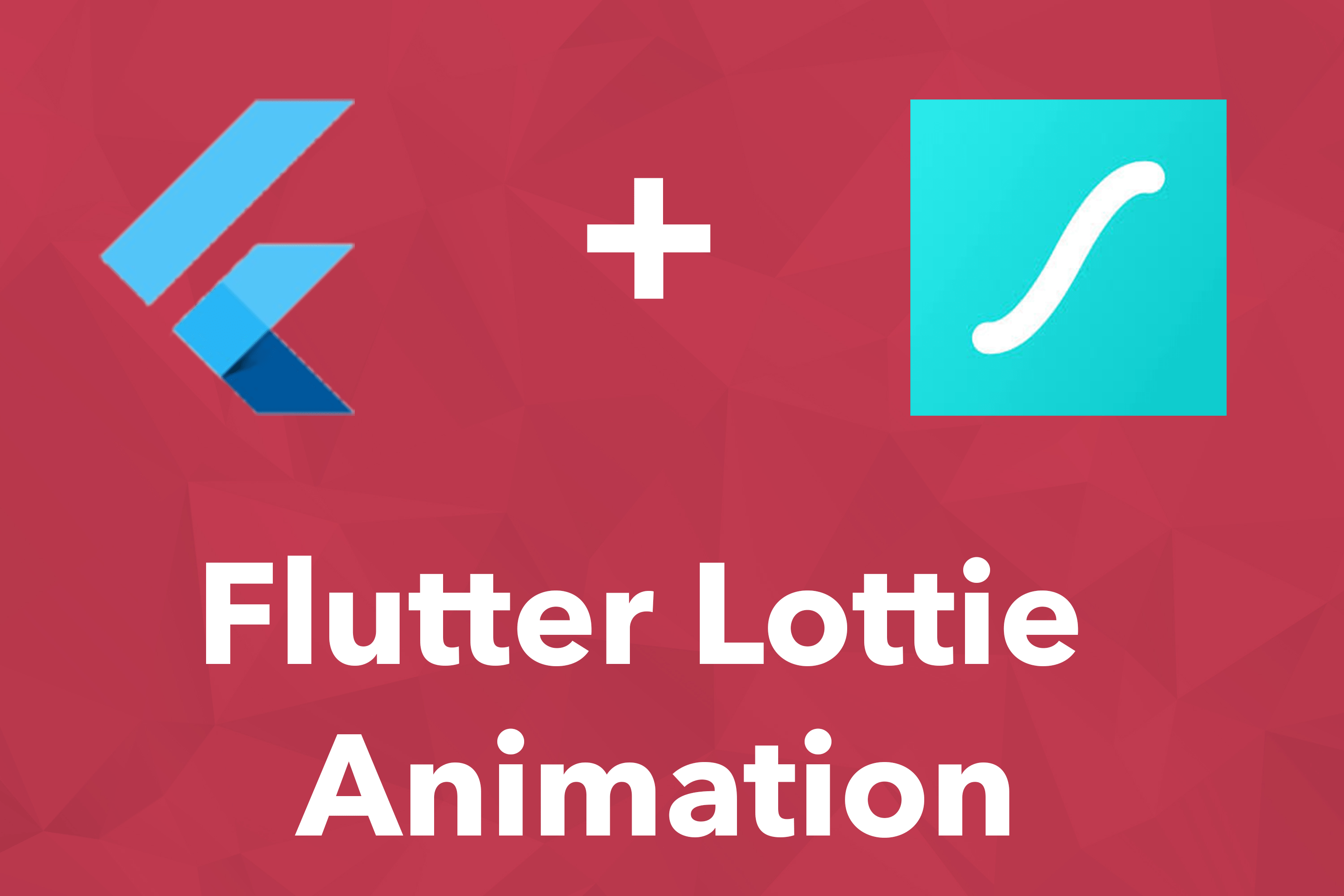
Flutter Lottie Animation
Why Lottie Library
Yes, mostly all beginners have the same question that why we use Lottie Animation when flutter provides a lot of animation widgets that more easy to use than Lottie animation.
Lottie is a widget who provides very cool animation who make the application more attractive, Lottie libraries and plugins available for free Web, iOS, Android, Flutter, React Native, Xamarin, Native Script, Windows, Vue, Angular, QT, Skia, Framer X, Sketch for free. We can easily get the Lottie animation file from https://lottiefiles.com and can use it our application.
In this article, I’ll show how to use Lottie widget.
1. Create a simple flutter project
2. add lottie_flutter in pubspec.yaml
lottie_flutter: ^0.2.0
3. Download this Lottie animation and put in <your-project>/assets
and mention in pubspec.yaml
assets: - assets/29-motorcycle.json
4. Import these below
import 'dart:async'; import 'dart:convert'; import 'dart:ui'; import 'package:lottie_flutter/lottie_flutter.dart'; import 'package:flutter/material.dart'; import 'package:flutter/services.dart' show rootBundle;
5. Main code of page on main.dart
class LottieDemo extends StatefulWidget { const LottieDemo({Key key}) : super(key: key); @override _LottieDemoState createState() => _LottieDemoState(); } class _LottieDemoState extends State<LottieDemo> with SingleTickerProviderStateMixin { LottieComposition _composition; AnimationController _controller; bool _repeat; @override void initState() { super.initState(); _repeat = true; _controller = AnimationController( duration: const Duration(milliseconds: 1), vsync: this, ); loadAsset("assets/29-motorcycle.json") .then((LottieComposition composition) { setState(() { _composition = composition; _controller.reset(); }); }); _controller.addListener(() => setState(() {})); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('Flutter Lottie Animation'), ), body: Container( color: Colors.white, child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Lottie( composition: _composition, size: const Size(300.0, 300.0), controller: _controller, ), Slider( value: _controller.value, onChanged: _composition != null ? (double val) => setState(() => _controller.value = val) : null, ), Row(mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ IconButton( icon: _controller.isAnimating ? const Icon(Icons.pause) : const Icon(Icons.play_arrow), onPressed: _controller.isCompleted || _composition == null ? null : () { setState(() { if (_controller.isAnimating) { _controller.stop(); } else { if (_repeat) { _controller.repeat(); } else { _controller.forward(); } } }); }, ), IconButton( icon: const Icon(Icons.stop), onPressed: _controller.isAnimating && _composition != null ? () { _controller.reset(); } : null, ), ]), ], ), ), ); } Future<LottieComposition> loadAsset(String assetName) async { return await rootBundle .loadString(assetName) .then<Map<String, dynamic>>((String data) => json.decode(data)) .then((Map<String, dynamic> map) => LottieComposition.fromMap(map)); } }
Output screen
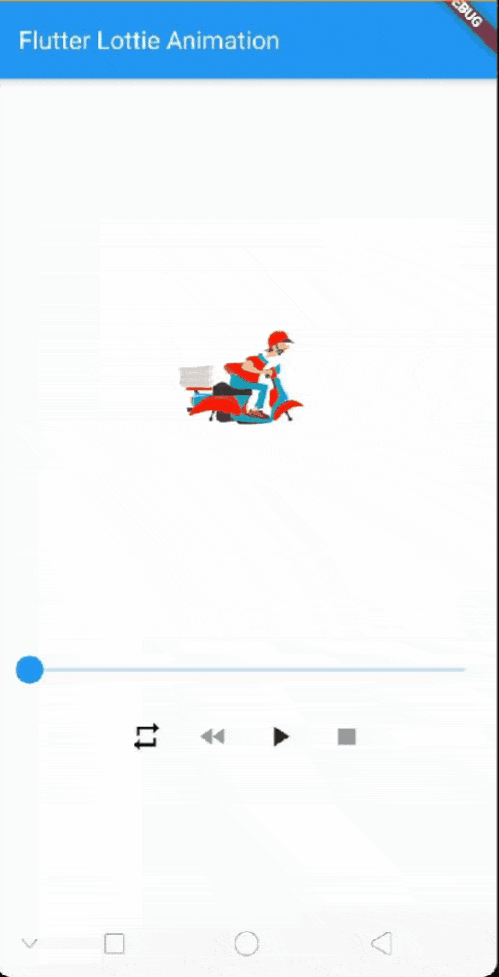
In same way you can get most used animation and add it in your app