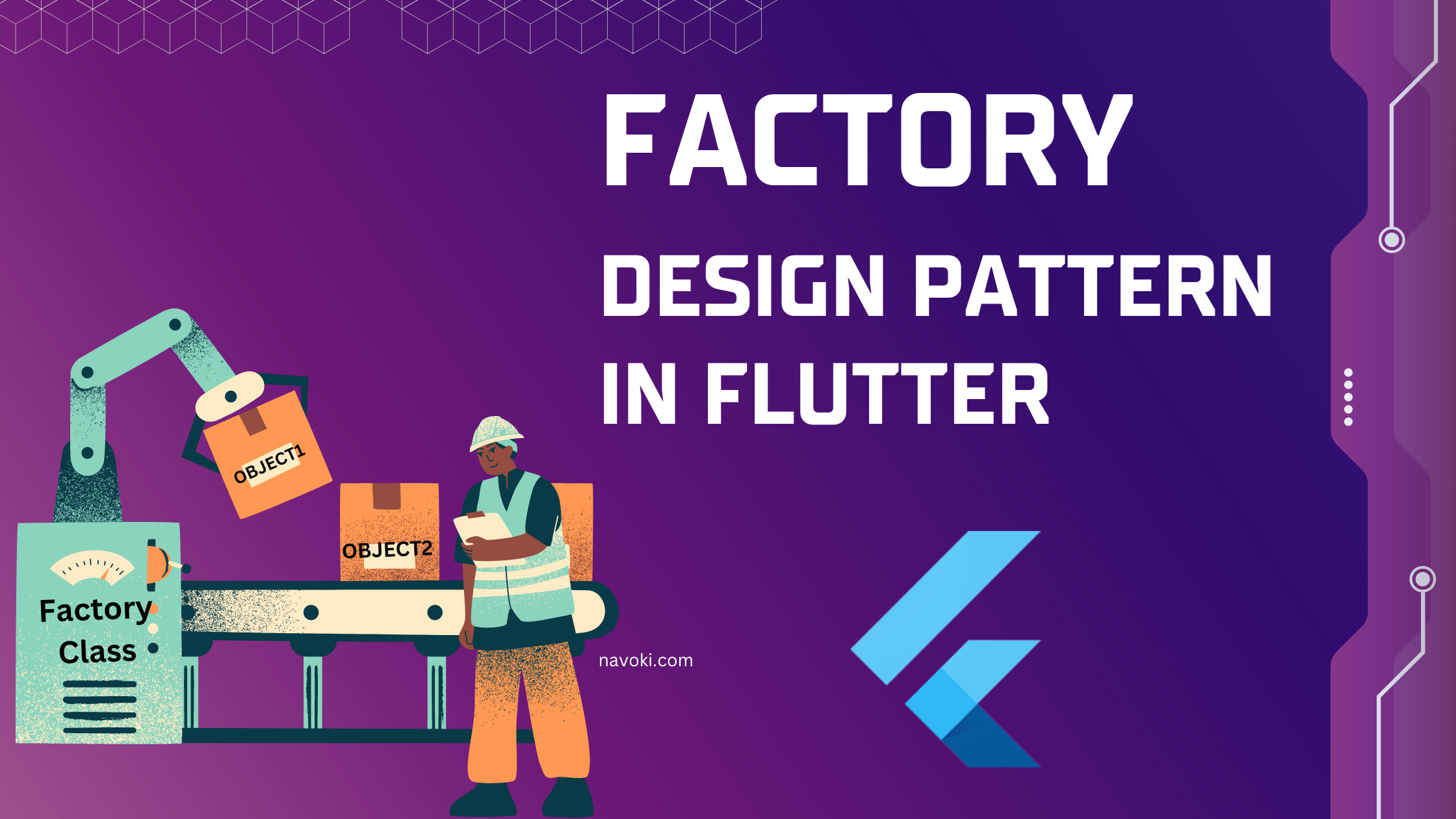
Expert Dart Programming: The Factory Design Pattern
The Factory Design Pattern is a smart way to make objects in programming, especially when you’re working with Dart to create Flutter apps. Instead of the usual way of making new objects (which is calling constructors), this pattern gives us a central place to handle object creation.
Why Should We Use the Factory Design Pattern for Flutter Apps?
Easy Management of Objects
For anyone developing Flutter apps, the Factory Design Pattern helps by putting all object creation in one place. This makes our code neater and easier to handle because there’s less repetition, and if we need to change how objects are made, we can do it in just one spot.
Keeping Things Separate
When building Flutter apps with Dart, using the Factory Design Pattern means the code that asks for new objects doesn’t need to know all the details about how those objects are made. This is good because it makes our app more flexible and easier to change later on.
When is the Factory Design Pattern Useful ?
This design pattern is really helpful when making new objects is complicated or needs special steps. By using this pattern, we can hide all that complexity away, which makes our Flutter app’s code cleaner and easier to work with.
A Real-World Example for Flutter Developers , Let’s think about a Flutter app that lets people pay in different ways, like with a Credit Card, UPI, or NetBanking. Here, the Factory Design Pattern can help us manage these payment options neatly. It allows us to keep our app’s code organized and easy to maintain, making life easier for anyone working with Dart and Flutter. This way, we can add new payment methods or change existing ones without causing a lot of headaches.
// Abstract class for Payment methods abstract class PaymentMethod { void doPayment(double amount); } // Concrete classes representing different payment methods class CreditCardPayment implements PaymentMethod { @override void doPayment(double amount) { print('Processing credit card payment of $amount'); } } class UpiPayment implements PaymentMethod { @override void doPayment(double amount) { print('Processing Upi payment of $amount'); } } class NetBankingPayment implements PaymentMethod { @override void doPayment(double amount) { print('Processing NetBanking payment of $amount'); } } // Factory class to create payment instances whichever user selects class PaymentMethodFactory { static PaymentMethod createPaymentMethod(String type) { switch (type) { case 'credit_card': return CreditCardPayment(); case 'upi': return UpiPayment(); case 'net_banking': return NetBankingPayment(); default: throw Argument Error('Invalid payment method'); } } } void main() { // Client code using the Factory Pattern, when user selects payment. final paymentMethod1 = PaymentMethodFactory.createPaymentMethod('upi'); paymentMethod1.doPayment(2500.0); // Output // Processing Upi payment of 2500.0 }
Follow Shivam Srivastava for Flutter and Mobile Dev related posts. LinkedIn, Instagram, Youtube
Join Discord for Flutter community https://bit.ly/48QaLey