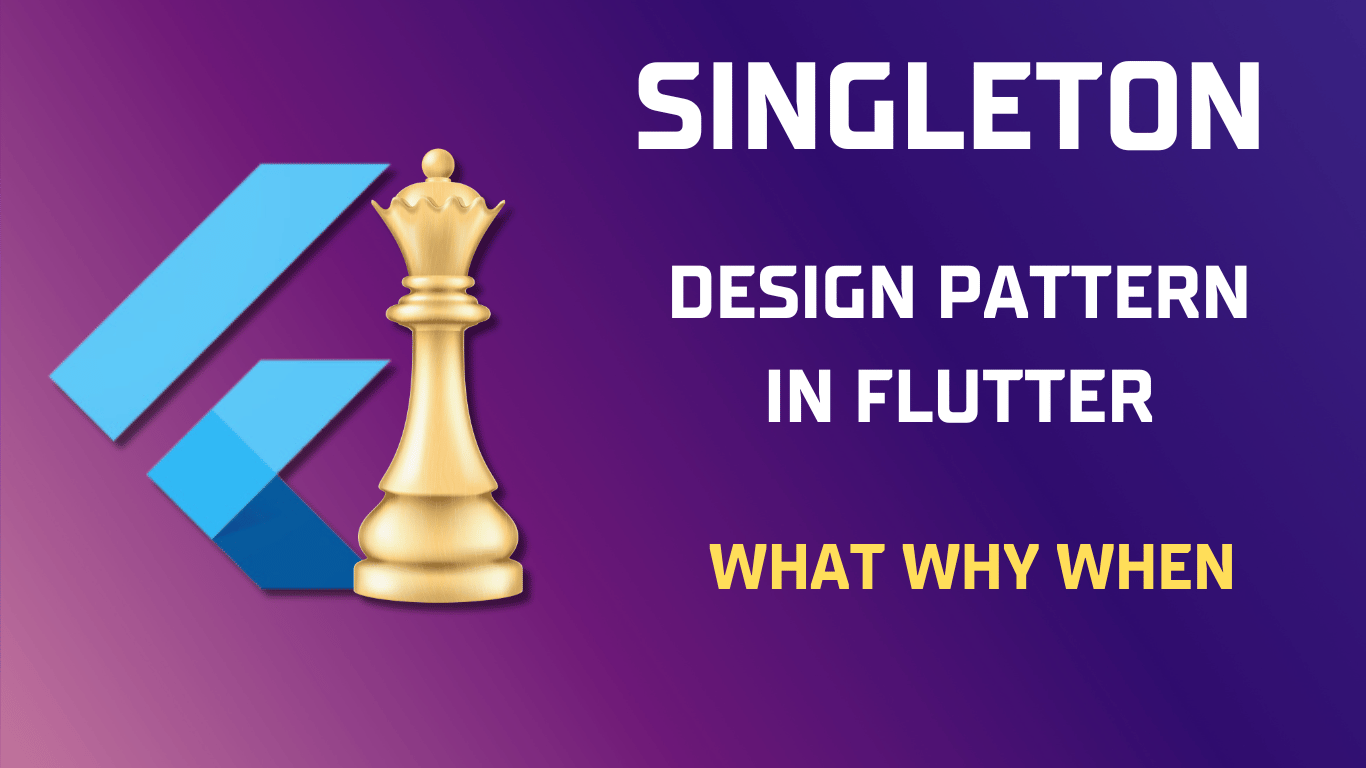
Expert Dart Programming: Singleton Design Pattern
In the ever-evolving world of mobile application development, Flutter has emerged as a leading framework, offering developers a seamless way to create visually stunning and functionally rich applications. Powered by the Dart programming language, Flutter allows for the creation of cross-platform apps with a single codebase, ensuring efficiency and consistency across iOS and Android platforms.
One of the fundamental concepts that every Flutter developer should be familiar with is the Singleton design pattern—a pattern that, while often used, may not always be fully understood in terms of its potential and application within the Flutter ecosystem.
Check Factory Design Pattern in Flutter
What is the Singleton Design Pattern?
At its core, the Singleton design pattern is a software design principle that ensures a class has only one instance and provides a global point of access to that instance. This means that no matter how many times you attempt to create a new instance of a class designated as a Singleton, you will always obtain the same, singular instance that was created initially.
Surprisingly, you might already be using this pattern in your Flutter apps without explicit awareness, given its ubiquitous nature in software development.
Why Use the Singleton Design Pattern?
The Singleton pattern is particularly beneficial in scenarios where controlled and global access to a shared resource is paramount. It encapsulates the instance creation mechanism, effectively restricting direct instantiation from outside the class. This controlled access ensures that the single instance can be globally accessed within the application, making it perfectly suited for managing shared resources across the entire app.
- Controlled Access: By encapsulating the instance creation, the Singleton pattern prevents unauthorized or unintended creation of multiple instances, ensuring that the critical resources are managed in a predictable manner.
- Global Access: Since the single instance is accessible globally within the application, it facilitates easy management of shared resources, from configuration settings to database connections, without the overhead of passing objects around or managing multiple instances.
When to Use the Singleton Design Pattern
The Singleton design pattern shines in several key scenarios within Flutter app development:
- Database Connections: To manage database connections efficiently, avoiding unnecessary multiple connections which can lead to resource exhaustion and data inconsistencies.
- Hardware Interface Access: Ideal for applications interacting with hardware resources such as audio players, printers, or file systems, ensuring synchronized access and operation.
- Service Objects: Perfect for services that offer functionality to various parts of the application, like third-party services, API clients, and more, providing a unified point of interaction.
Alternatives to Singleton in Flutter
While the Singleton pattern offers numerous advantages, it’s not without its critics, particularly concerning testing and scalability. As an alternative, Dependency Injection (DI) has gained popularity for managing object lifecycles and ensuring that a single instance of a class is created and used where needed. DI frameworks offer a more flexible and decoupled way to achieve similar goals as the Singleton pattern, promoting easier testing and maintenance.
Example: Singleton in Flutter
Implementing the Singleton pattern in Flutter using Dart is straightforward. Here’s a simplified example:
AppConfig will be a our Object to be shared across app
class AppConfig { final String baseUrl; AppConfig({required this.baseUrl}); void printBaseUrl() { print(baseUrl); } }
AppConfigProvider uses InheritedWidget, so we can provide instance along the widget tree
class AppConfigProvider extends InheritedWidget { final AppConfig config; const AppConfigProvider({ Key? key, required this.config, required Widget child, }) : super(key: key, child: child); @override bool updateShouldNotify(AppConfigProvider oldWidget) { return config != oldWidget.config; } static AppConfig? of(BuildContext context) { return context.dependOnInheritedWidgetOfExactType<AppConfigProvider>()?.config; } }
Since we are using InheritedWidget at MaterialApp level, the object will be available across app, down the widget tree.
void main() { var appConfig = AppConfig(baseUrl: "https://example.in"); runApp(AppConfigProvider(config: appConfig, child: MyApp())); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { final appConfig = AppConfigProvider.of(context); return MaterialApp( title: appConfig?.baseUrl ?? "Default Url", home: Scaffold( appBar: AppBar( title: Text(appConfig?.baseUrl ?? "Missing Config"), ), body: Center( child: TextButton( onPressed: () => appConfig?.printBaseUrl(), child: Text("Print App Url"), ), ), ), ); } }
Conclusion
The Singleton design pattern is a powerful tool in the arsenal of a Flutter developer. By providing controlled and global access to shared resources, it helps maintain application consistency and efficiency. Whether you’re managing database connections or integrating third-party services, understanding when and how to implement the Singleton pattern can significantly enhance your Flutter app’s architecture.
However, it’s also crucial to consider alternatives like Dependency Injection to ensure your app remains flexible, testable, and scalable as it grows.
Check Factory Design Pattern in Flutter
Follow Shivam Srivastava for Flutter and Mobile Dev related posts. LinkedIn, Instagram, Youtube