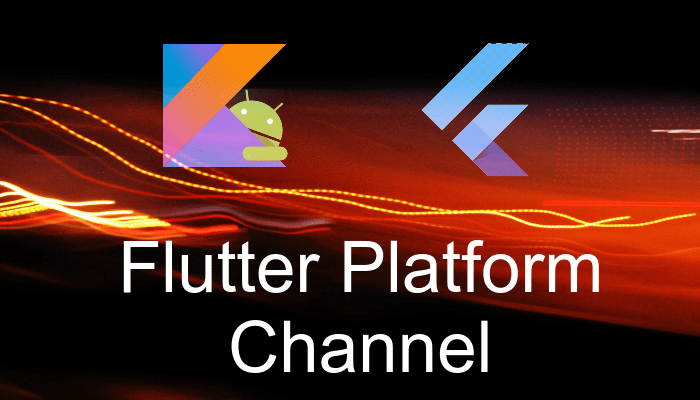
Flutter Platform Channel
- Posted by Shivam Srivastava
- Categories Flutter
- Date April 1, 2020
Introduction
Flutter permits us to call platform-specific APIs accessible in Kotlin or Java code on Android and in Swift or Objective C code on iOS. Flutter’s platform-specific API works with message passing.
From the Flutter app, we’ve to send messages to a bunch on iOS or Android elements of the app over a platform channel. The host listens on the platform channel and gets the message. It then uses any platform-specific APIs applying the native programming language and sends back a response to the Flutter portion of the application. Messages are passed b/w the Flutter Code(UI) and host (platform) using platform channels. Messages and responses are passed asynchronously and therefore the interface remains responsive.
On Dart side victimization Method Channel(API) we send a message that is corresponding a method call. On the Android side Method Channel Android (API) and on the iOS side FlutterMessageChannel (API) is applied for receiving method calls and sending back the result. These classes enable us to develop a platform plugin with an easily understandable and simple code.
If needed, method calls can be sent within the opposite direction, with the IOS/Android platform acting as client and method implemented in Dart.
Architecture overview
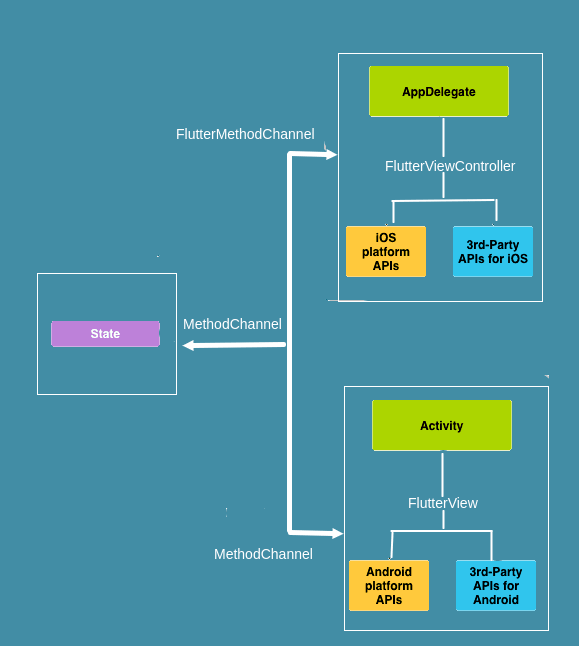
Purpose of this article?
As we know the use of Platform Channel, So in this article, I will explain how to Use Platform channel with Android applications with some basic examples. As you know that we can use Java or Kotlin for Android, so I will use Kotlin language you can use Java also.
I will explain below 2 examples with code in this article.
- Sum of two numbers given by the user.
- Open URL in the browser.
Let’s Begin
If you don’t have a flutter set up in your machine so you have to need to install it first. Click here to learn how to set up flutter on Windows and for Linux click here.
Get Ready
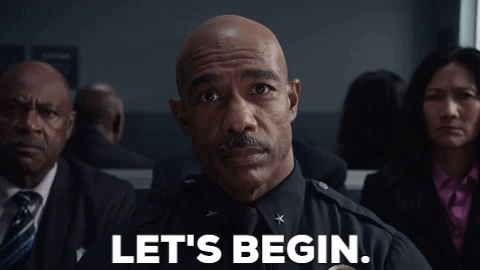
Create a platform Channel
Create a platform channel as given below code, remember all the platform channel in the same app should be unique else you will get an error, in my case my channel name is “navoki” which is passed in MethodChannel Constructor.
static const platform = const MethodChannel('navoki');
Invoke method on platform Channel
First, we’re Invoking a method on the method channel, defining the concrete method to call via the String identifier. Below I’m calling two different functions which are some of two numbers and the other one is open URL in a browser.
Future<String> _platformChannelSum( String firstNumber, String secondNumber) async { var sendMap = <String, String>{ 'firstNumber': firstNumber, 'secondNumber': secondNumber }; String value = ""; try { value = await platform.invokeMethod('getMessage', sendMap); } catch (e) { print(e); } return value; }
Future<void> _openUrl() async { try { await platform.invokeMethod('openUrl'); } catch (e) { print(e); } }
Create method implementation in Android using Kotlin
Open MainActivity.kt file in the Android folder and create a MethodChannel with the same name that we have created in Flutter App as the name of “navoki” (in my case).
class MainActivity : FlutterActivity() { String methodChannelName="navoki";
Create a MethodCallHandler in onCreate method
MethodChannel(flutterView, methodChannelName).setMethodCallHandler { methodCall, result -> val arguments = methodCall.arguments<Map<String, String>>() if (methodCall.method == "getMessage") { val firstNumber = arguments["firstNumber"] as String val secondNumber = arguments["secondNumber"] as String val f = firstNumber.toInt() val s = secondNumber.toInt() val message = "$firstNumber + $secondNumber = ${firstNumber.toInt() + secondNumber.toInt()}" result.success(message) }else{ val webpage = Uri.parse("https://navoki.com") val intent = Intent(Intent.ACTION_VIEW, webpage) if (intent.resolveActivity(packageManager) != null) { startActivity(intent) } } }
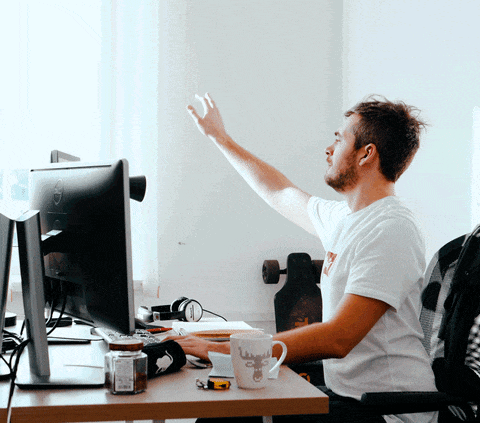
Get the complete source code on Github.
Senior Tech Lead at Citridot , Android and Flutter Dev, Dart ,Founder of Navoki
You may also like
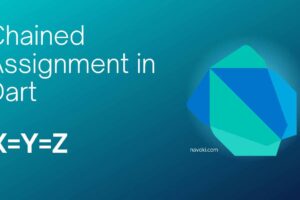
The Chain Assignment Trick : Dart Programming
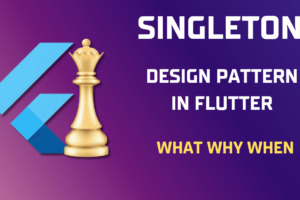
Expert Dart Programming: Singleton Design Pattern
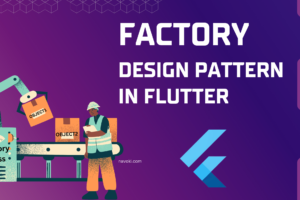