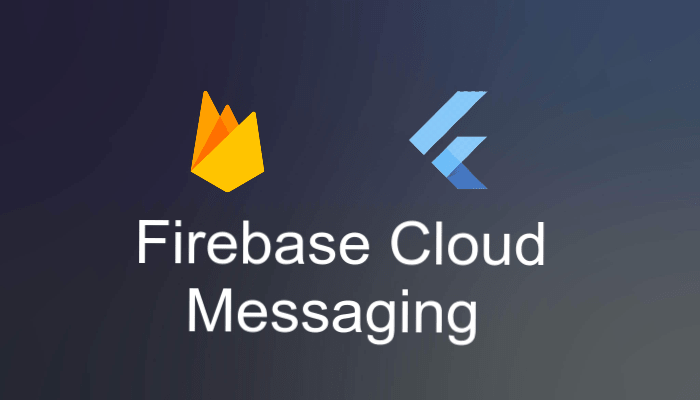
Firebase Cloud Messaging Using Flutter
What is Firebase Cloud Messaging
Firebase Cloud Messaging (FCM), erst known as Google Cloud Messaging (GCM), could be a free cloud service from Google that enables app developers to send notifications and messages to users across a different platform, including with Android, iOS and Web applications.
Purpose of this article?
In this Flutter tutorial, we’ll show you ways to integrate Firebase Cloud Messaging (FCM) push notification to Android Apps, we’ll use firebase_messaging plugin.
Setup Flutter SDK
If you don’t have a flutter set up in your machine so you have to need to install it first. Click here to learn how to set up flutter on Windows and for Linux click here.
Create a Firebase Project
For use firebase properties you have to need to register your app in firebase. First, integrate your flutter project to firebase, if you don’t know how to do it, read my Flutter Firebase Integration article.
- Add the below code in AndroidMenifest.xml.
<intent-filter> <action android:name="FLUTTER_NOTIFICATION_CLICK" /> <category android:name="android.intent.category.DEFAULT" /> </intent-filter>
- Add firebase_messaging dependency in your project pubspec.yaml file.
dependencies: flutter: sdk: flutter firebase_messaging: ^6.0.13
- Add the below code in your main.dart file for import dependency.
import 'package:firebase_messaging/firebase_messaging.dart';
void firebaseCloudMessaging_Listeners() { _firebaseMessaging.getToken().then((token) { print(token); }); try { _firebaseMessaging.configure( onMessage: (Map<String, dynamic> message) async { print("onMessage: $message"); final notification = message['notification']; print(notification); }, onLaunch: (Map<String, dynamic> message) async { print("onLaunch: $message"); final notification = message['data']; print(notification); }, onResume: (Map<String, dynamic> message) async { print("onResume: $message"); final notification = message['data']; print(notification); }, ); _firebaseMessaging.requestNotificationPermissions( const IosNotificationSettings(sound: true, badge: true, alert: true)); } catch (e) { print('Error----$e'); } }
We have done all programming, now we are testing it using push notification from firebase console.
- Go to firebase console -> grow -> cloud messaging.
- Click send your first message.
- Enter Notification Title and body text.
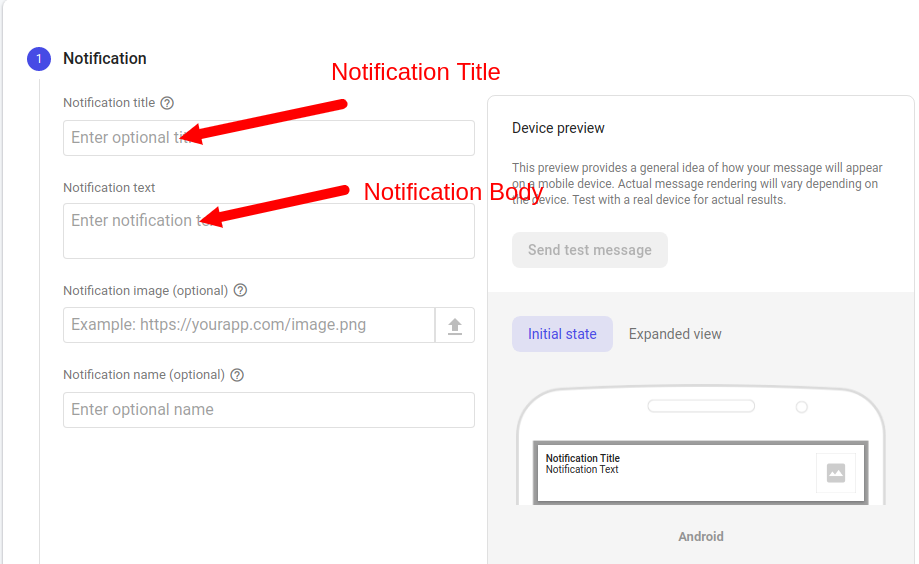
- Select an app
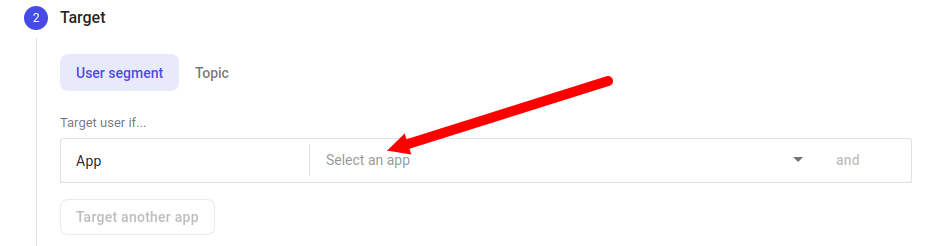
- Schedule notification delivered time.
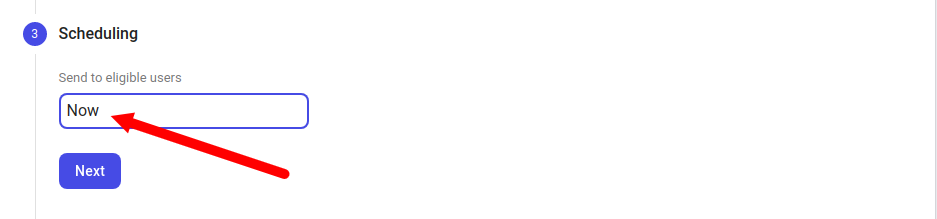
- Set the listener and body parameter of notification. Key click_action and it’s value FLUTTER_NOTIFICATION_CLICK should be the same as given in the below image but another parameter can be a change, if you update the parameter’s key then don’t forget to update the key in code also.
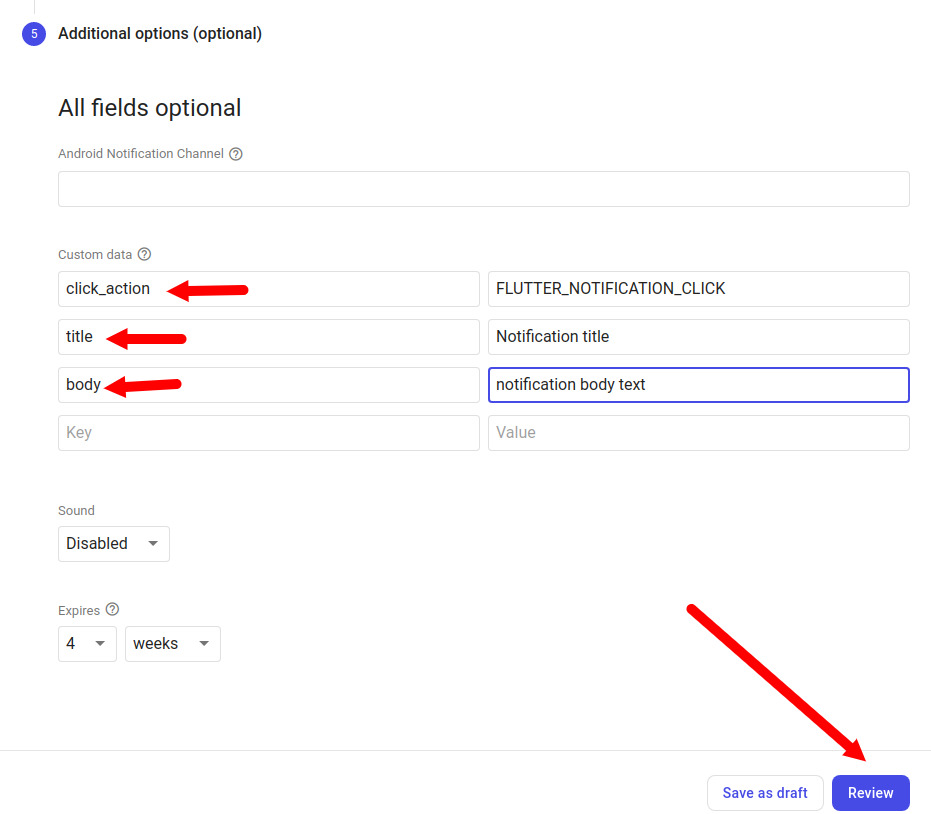
- Now we have done everything, now just click on the review button and publish it.
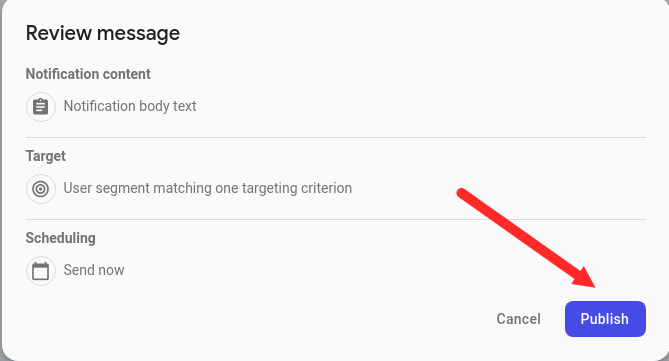
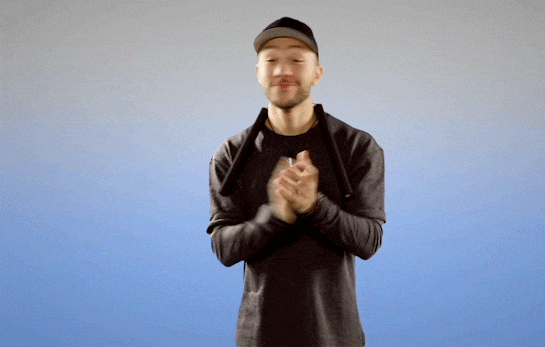
Get complete source code