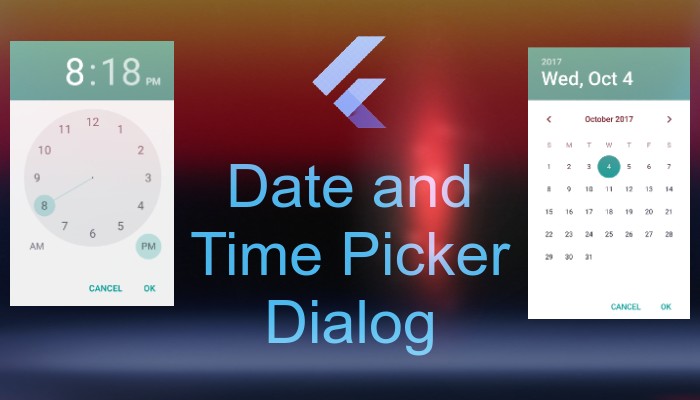
DATE TIME PICKER DIALOG USING FLUTTER
Introduction
In this article, we will add Date and Time picker in flutter application without any plugin.
Purpose of this article?
In this tutorial, you’ll build a mobile app that includes a DateTime Picker Dialog using the Flutter SDK. Your app will:
- Display Date and Time picker separately.
- Display the selected data as outputs in Text Widgets.
In this tutorial, we will focus on adding a DateTime Picker Dialog in your flutter application.
Setup Flutter on your machine
If you don’t have a flutter set up in your machine so you have to need to install it first. Click here to learn how to set up flutter on Windows and for Linux click here.
Let’s Begin for the code.
- Add intl dependency in your project pubspec.yaml file.
dependencies: flutter: sdk: flutter intl: <latest version>
intl: This dependency set format of choose selected date or time.
Run flutter packages get command on terminal- Add the below code in your main.dart file for import dependency.
import 'package:intl/intl.dart';
Now we are ready for Coding the component.
Show Date Picker Dialog.
Future<void> _showDatePicker()async{ final DateTime picked=await showDatePicker(context: context, initialDate: DateTime.now(), firstDate: DateTime(2015, 8), lastDate: DateTime(2101)); if(picked != null) { print(DateFormat("yyyy-MM-dd").format(picked)); } }
Using this code a simple calendar arises on a screen in a dialog box.
Show Time Picker Dialog.
Future<void> _showTimePicker()async{ final TimeOfDay picked=await showTimePicker(context: context,initialTime: TimeOfDay(hour: 5,minute: 10)); if(picked != null) { print(picked.format(context)); } }
Using this code a simple time chooser arises on a screen in a circle dialog box.
Show Date and Time Picker Dialog.
Future<void> _showDateTimePicker()async { final DateTime datePicked=await showDatePicker(context: context, initialDate: DateTime.now(), firstDate: DateTime(2015, 8), lastDate: DateTime(2101)); if(datePicked!=null) { final TimeOfDay timePicked=await showTimePicker(context: context,initialTime: TimeOfDay(hour: TimeOfDay.now().hour,minute: TimeOfDay.now().minute)); if(timePicked !=null) { print("${ DateFormat("yyyy-MM-dd").format(datePicked)} ${timePicked.format(context)}"); } } }
Using this code you first get a simple calendar for choosing a date then after you get a simple Time picker in circle dialog watch.
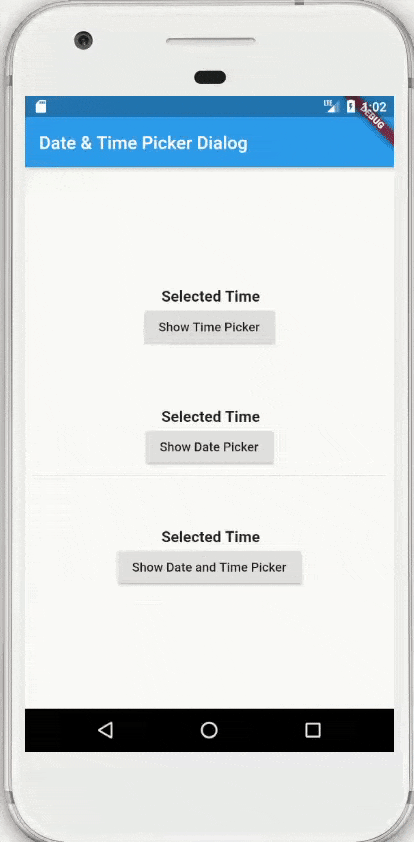
You can find given example’s complete code on my GitHub repo click here.