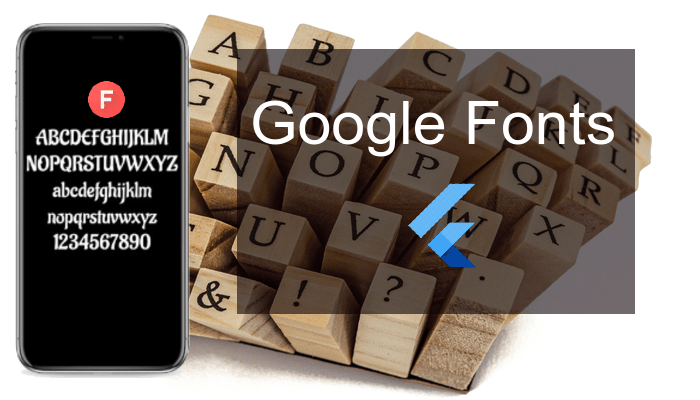
Flutter Google Fonts
Introduction
In recent months Google has announced the library of Google fonts, who helps developers a lot to modify the font of Flutter Text. The google_fonts
package for Flutter allows you to easily use any of the 977 fonts (and their variants) from fonts.google.com in your Flutter app. The big thing is that you don’t have a need to add any .ttf or .otf file in the asset folder and mapped it with pubspec.yaml file. As I have already written another blog also about Flutter custom font, where you have to need to add your font file manually in the assets folder and mapped it with pubspec.yaml file.
In this article, I’ll show you how to implement the google_fonts library in your flutter project and how to use the different fonts in it.
Let’s begin
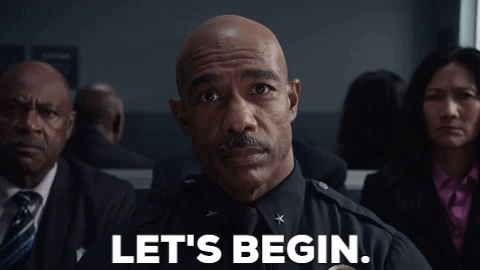
Add dependency
First, you have a need to add a google_fonts
library in your pubspec.yaml file.
dependencies: flutter: sdk: flutter google_fonts: <latest-version>
Import files
import 'package:google_fonts/google_fonts.dart';
Now we are ready to code for UI components. I am using simple Text Widgets. You can also use other properties of the Text Widget as you used in TextStyle, it will not reflect your font and work fine. There have a many ways to apply google fonts in your flutter projects, some examples are given below.
To use GoogleFonts
with the default TextStyle:
Text( "Hello World", style: GoogleFonts.astloch(fontSize: 20), ),
To use GoogleFonts
dynamically
Text( 'Hello World', style: GoogleFonts.getFont('Lato'), ),
To use GoogleFonts
with an existing TextStyle
:
Text( 'Hello World', style: GoogleFonts.lato( textStyle: TextStyle(color: Colors.green), ), ),
To use GoogleFonts
with Theme
Text( 'Hello World', style: GoogleFonts.lato(textStyle: Theme.of(context).textTheme.display1), ),
To override the properties
Text( 'Hello World', style: GoogleFonts.lato( textStyle: Theme.of(context).textTheme.display1, fontSize: 20 fontWeight: FontWeight.bold fontStyle: FontStyle.italic, ), ),
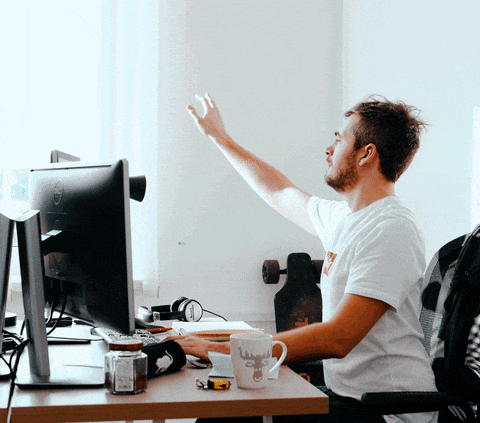