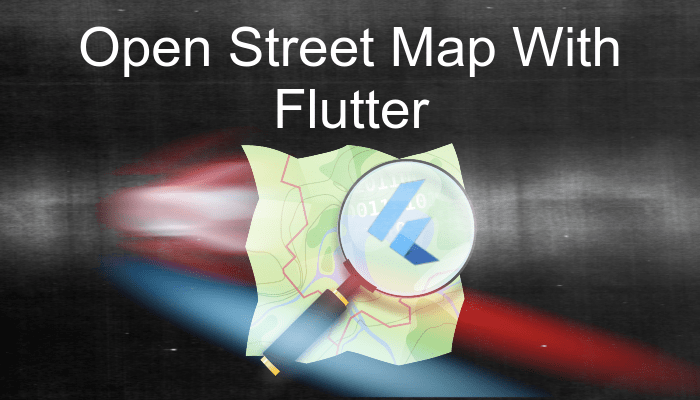
Open Street Map Using Flutter
Introduction
According to the Open Street Map Community, OpenStreetMap (OSM) is a cooperative project to make a free editable map of the Earth. … the information from OpenStreetMap will be used in varied ways that together with the production of paper maps and electronic maps (i.e. Google Maps), geocoding of address and place names, and route designing.
Purpose of this article?
As you may have understood from the title of this article that we will discuss how to integrate Open Street Map In Flutter. The Open Street Map is one of the map like Google Map, so follow all the below steps in sequence as given to integrating OSM in your flutter application successfully.
Setup Flutter SDK
If you don’t have a flutter set up in your machine so you have to need to install it first. Click here to learn how to set up flutter on Windows and for Linux click here.
Add Dependencies
- Add flutter_osm_plugin dependency in your project pubspec.yaml file.
dependencies: flutter: sdk: flutter flutter_osm_plugin: <latest_version>
Run flutter packages get command on terminal
- Add the below code in your main.dart file for import dependency.
import 'package:flutter_osm_plugin/flutter_osm_plugin.dart';
Now we are ready to code the components.
GlobalKey<OSMFlutterState> mapKey = GlobalKey<OSMFlutterState>();
- Get current location latitude and longitude using the below code.
getMyLocation()async { try { GeoPoint p = await mapKey.currentState.myLocation(); double lat=p.latitude; double lon=p.longitude; print( "latitude is :$lat, longitude is:$lon"); } on GeoPointException catch (e) { print( "${e.errorMessage()}"); } }
- Draw Road between two points using the below code.
drawRoadOnMap()async { try { await mapKey.currentState.drawRoad( GeoPoint(latitude: 28.596429, longitude: 77.190628), GeoPoint(latitude: 28.4573802, longitude: 73.1424312)); } on RoadException catch (e) { print( "${e.errorMessage()}"); } }
- Add the OSMFlutter widget in your UI part.
OSMFlutter( key: mapKey, currentLocation: true, road: Road( startIcon: MarkerIcon( icon: Icon( Icons.person, size: 64, color: Colors.brown, ), ), roadColor: Colors.blueAccent), markerIcon: MarkerIcon( icon: Icon( Icons.person_pin_circle, color: Colors.redAccent, size: 56, ), ), initPosition: GeoPoint(latitude: 28.4573802, longitude: 73.1424312), useSecureURL: false, ),
output:
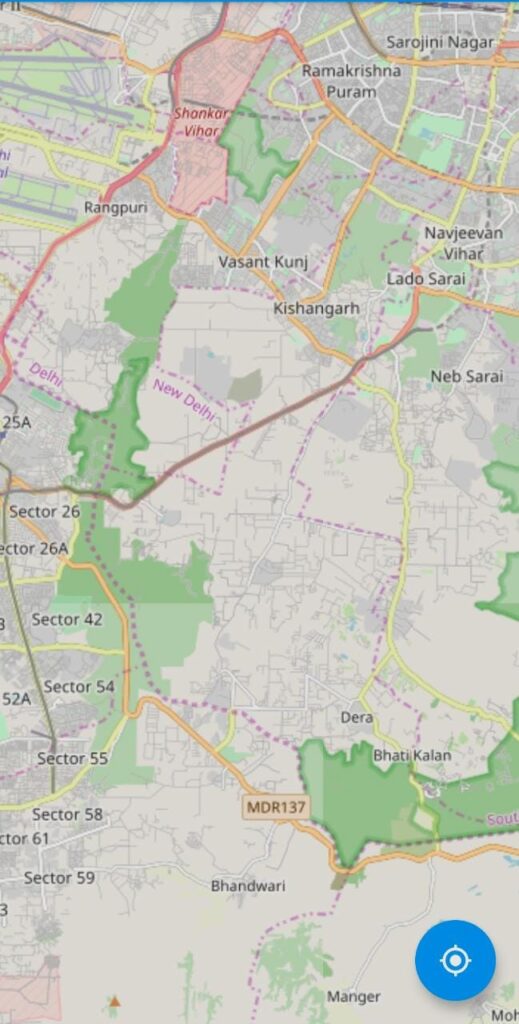
Get Complete source code.