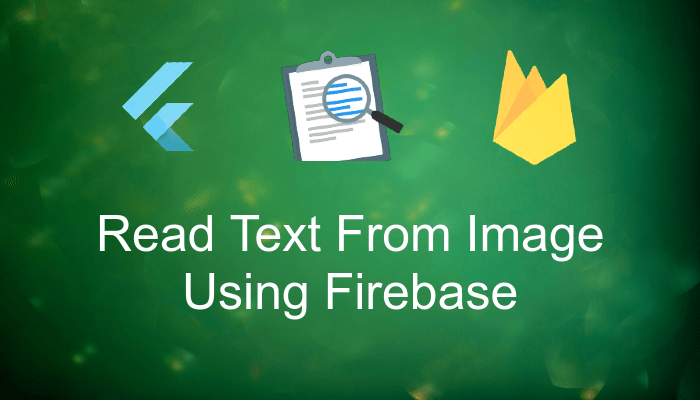
DETECT TEXT FROM IMAGE USING FIREBASE
Introduction
So, I know Machine Learning is there in open and it’s been there for quite long. It goes means back in the previous century once it had been initially coined and brought into existence, currently, you discover it every place in existence therefore vital that your life is perhaps enclosed by it and you will even not understand, consider your smartphones they’re referred to as “smart” for a specific reason and nowadays they’re “smartest” while getting even more n more from day to day, as a result of they’re learning regarding you from you itself the additional you’re using it the more your smartphone gets to grasp you and every one of that’s simply the machine with lines of code evolving with time like humans. It’s not simply restricted to smartphones, it’s in-depth in technology.
Purpose of this article?
Nowadays every developer familiar with the term Machine Learning In this article, today we create a simple text detector from image application using Flutter and Firebase ML kit, also I explain all the steps in a simple manner so anybody can use the Firebase ML kit in a flutter project.
If you don’t have a flutter set up in your machine so you have to need to install it first. Click here to learn how to set up flutter on Windows and for Linux click here.
Create a Firebase Project
For use firebase properties you have to need to register your app in firebase. First, integrate your flutter project to firebase, if you don’t know how to do it, read my Flutter Firebase Integration article.
Let’s Begin for the code.
- Add firebase_ml_vision and image_picker dependency in your project pubspec.yaml file.
dependencies: flutter: sdk: flutter firebase_ml_vision: <latest_version> image_picker: <latest_version> translator: <latest_version>
firebase_ml_vision: This dependency used for access Firebase ML kit.
image_picker: This dependency used to pick an image.
translator: This dependency used to convert the text into your language.
- Add the below code in your main.dart file for import dependency.
import 'package:image_picker/image_picker.dart'; import 'package:firebase_ml_vision/firebase_ml_vision.dart'; import 'package:translator/translator.dart';
Now we are ready for Coding the component.
Now we are ready to code the components, as in the below code I am taking image from the gallery, you can get from the camera also as you need, after getting the image we store all the text in List.
Creating a variable which we have needed for code.
bool _isImageLoaded = false; bool _isTextLoaded = false; String _text = ""; File _pickedImage;
Future<void> geTextImage() async { var tempStore = await ImagePicker.pickImage(source: ImageSource.gallery); setState(() { _pickedImage = tempStore; _isImageLoaded = true; }); FirebaseVisionImage ourImage = FirebaseVisionImage.fromFile(_pickedImage); TextRecognizer recognizeText = FirebaseVision.instance.textRecognizer(); VisionText readText = await recognizeText.processImage(ourImage); GoogleTranslator translator = GoogleTranslator(); String input = readText.text; translator.translate(input, to: 'en').then((_translatedText) => { setState(() { _text = _translatedText; _isTextLoaded = true; }) }); }
Now call this function in your flutter application the same as calling other functions. You can modify the text language as you need, for change the language changes the parameter value “en” to another. i.e. to convert the text language write “hi” instead of “en”.
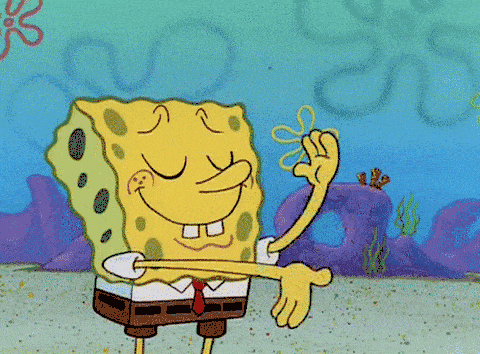