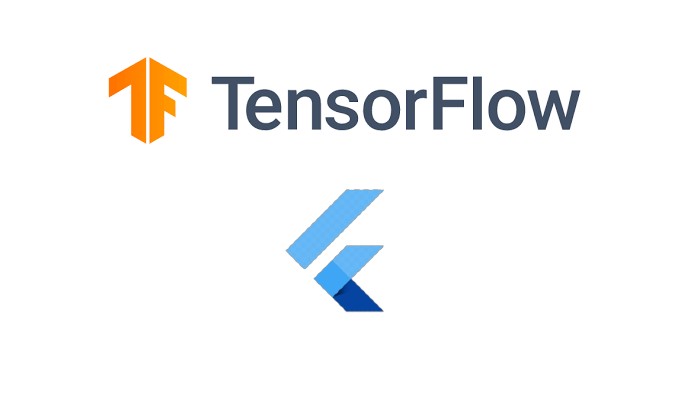
Objects Detection From Image Using Flutter
Introduction
So, we know Machine Learning is there in open and it’s been there for quite long. It goes means back in the previous century once it had been initially coined and brought into existence, currently, you discover it every place in existence therefore vital that your life is perhaps enclosed by it and you will even not understand, consider your smartphones they’re referred to as “smart” for a specific reason and nowadays they’re “smartest” while getting even more n more from day to day, as a result of they’re learning regarding you from you itself the additional you’re using it the more your smartphone gets to grasp you and every one of that’s simply the machine with lines of code evolving with time like humans. It’s not simply restricted to smartphones, it’s in-depth in technology.
Purpose of this article?
In this blog, we will discuss one of the most important concepts of machine learning that is to Detect Objects from images using flutter. We can Detect objects using different models like MobileNet, SSD, DeepLab, YOLOv2, etc. but in this blog, we will see only YOLO and SSD then rest will discuss in upcoming articles.
What is Object Detection
Object detection is one among the classical issues in pc vision wherever you’re working to recognize what and wherever — specifically what objects are within a given image and also wherever they’re within the image. The matter of object detection is a lot of complicated than classification, which can also acknowledge objects but doesn’t indicate where the object is found within the image. Additionally, the classification doesn’t work on pictures containing more than one object.
What is YOLO?
YOLO stands for “You Only Look Once”, this is a real-time object detection algorithm, that is one of the most effective object detection algorithms that also encompasses many of the foremost innovative concepts beginning of the pc vision analysis community.
What is SSD
SSD stands for Single Shot Multi-Box Detector, this is a famous algorithm in object detection whereas Mobile net is a convolution neural network accustomed to turning out high-level options.
Let’s Begin
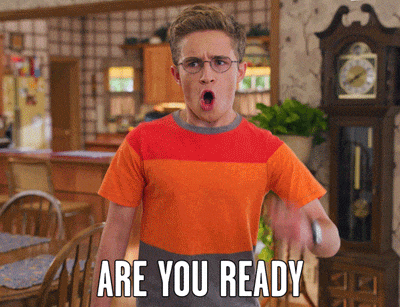
- Add tflite and image_picker dependency in your project pubspec.yaml file.
dependencies: flutter: sdk: flutter tflite: ^1.0.4 image_picker: ^0.6.1+8
tflite: This dependency used to access ML objects.
image_picker: This dependency used to get a barcode image.
- Create a
assets
folder and place your label file and model file in it. Inpubspec.yaml
, you can download files from here.
assets: - assets/ssd_mobilenet.tflite - assets/ssd_mobilenet.txt - assets/yolov2_tiny.tflite - assets/yolov2_tiny.txt
- In android/app/build.gradle add the following setting in
android
block.
aaptOptions { noCompress 'tflite' noCompress 'lite' }
- Add the below code in your main.dart file for import dependency.
import 'package:tflite/tflite.dart'; import 'package:image_picker/image_picker.dart';
Now we are ready for Coding the component.
const String ssd = "SSD MobileNet"; const String yolo = "Tiny YOLOv2"; String _model = ssd; File _image; Color objectAreaColor=Colors.red; double _imageWidth; double _imageHeight; bool _busy = false; List _recognitions;
pickImageFromGallery() async { var image = await ImagePicker.pickImage(source: ImageSource.gallery); if (image == null) return; setState(() { _busy = true; }); predictImage(image); }
We have done code to pick the image, now we are checking which model should apply on the image and then get the objects.
predictImage(File image) async { if (image == null) return; if (_model == yolo) { await yolov2Tiny(image); } else { await ssdMobileNet(image); } FileImage(image) .resolve(ImageConfiguration()) .addListener((ImageStreamListener((ImageInfo info, bool b) { setState(() { _imageWidth = info.image.width.toDouble(); _imageHeight = info.image.height.toDouble(); }); }))); setState(() { _image = image; _busy = false; }); }
yolov2Tiny(File image) async { var recognitions = await Tflite.detectObjectOnImage( path: image.path, model: "YOLO", threshold: 0.3, imageMean: 0.1, imageStd: 255.0, numResultsPerClass: 1); setState(() { _recognitions = recognitions; }); }
ssdMobileNet(File image) async { var recognitions = await Tflite.detectObjectOnImage( path: image.path, numResultsPerClass: 1); setState(() { _recognitions = recognitions; }); }
Now We are getting the size of objects and the show it as a border.
List<Widget> renderBoxes(Size screen) { if (_recognitions == null) return []; if (_imageWidth == null || _imageHeight == null) return []; double factorX = screen.width; double factorY = _imageHeight / _imageHeight * screen.width; Color areaColor = objectAreaColor; return _recognitions.map((re) { return Positioned( left: re["rect"]["x"] * factorX, top: re["rect"]["y"] * factorY, width: re["rect"]["w"] * factorX, height: re["rect"]["h"] * factorY, child: Container( decoration: BoxDecoration( border: Border.all( color: areaColor, width: 3, )), child: Text( "${re["detectedClass"]} ${(re["confidenceInClass"] * 100).toStringAsFixed(0)}%", style: TextStyle( background: Paint()..color = areaColor, color: Colors.white, fontSize: 15, ), ), ), ); }).toList(); }
Now, loading the model, this is the most important thing so write the code carefully or you can copy the code.
@override void initState() { super.initState(); _isBusy = true; loadModel().then((val) { setState(() { _isBusy = false; }); }); } loadModel() async { Tflite.close(); try { String res; if (_model == yolo) { res = await Tflite.loadModel( model: "assets/yolov2_tiny.tflite", labels: "assets/yolov2_tiny.txt", ); } else { res = await Tflite.loadModel( model: "assets/ssd_mobilenet.tflite", labels: "assets/ssd_mobilenet.txt", ); } print(res); } on PlatformException { print("Something happens wrong"); } }
We have done all the necessary stuff, now we design the layout and call the function as need.
Stack( children: <Widget>[ Positioned( top: 0.0, left: 0.0, width: size.width, child: _image == null ? Align(alignment:Alignment.center,child: Text("No Image Selected")) : Image.file(_image), ), Align( alignment: Alignment.bottomCenter, child: Row( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: <Widget>[ RaisedButton(onPressed: (){ if(_model==yolo) { setState(() async { _model = ssd; loadModel(); await predictImage(_image); objectAreaColor=Colors.blue; }); } else { setState(() async { _model = yolo; loadModel(); await predictImage(_image); objectAreaColor=Colors.red; }); } },child: Text("Change Model"),), RaisedButton(onPressed: pickImageFromGallery, child: Text("Select Image"),), ], ), ), if(_isBusy) Center( child: CircularProgressIndicator(), ) ]..addAll(renderBoxes(size)), ),
Get the complete source code.