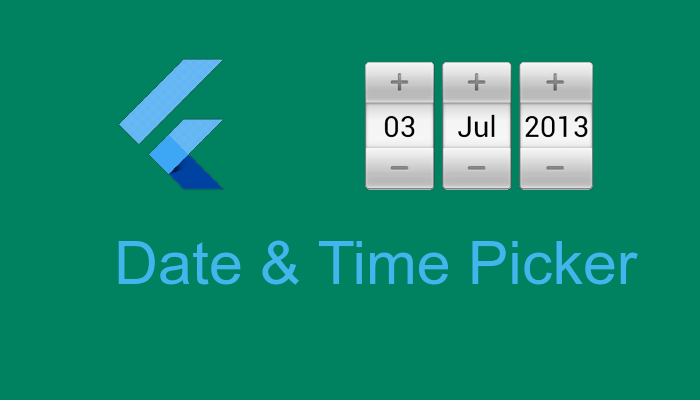
DATE TIME PICKER USING FLUTTER
Introduction
Using Flutter Date Time Picker Plugin you can add Date and Time picker in your flutter application.
What do you learn here?
In this tutorial, you’ll build a mobile app that includes a DateTime Picker using the Flutter SDK. Your app will:
- Display Date and Time picker separately.
- Display the selected data as outputs in Text Widgets.
In this tutorial, we will focus on adding a Date_Time Picker in your flutter application and.
Setup Flutter on your machine
If you don’t have a flutter set up in your machine so you have to need to install it first. Click here to learn how to set up flutter on Windows and for Linux click here.
Let’s Begin for the code.
- Add flutter_datetime_picker and intl dependency in your project pubspec.yaml file.
dependencies: flutter: sdk: flutter flutter_datetime_picker: <latest version> intl: <latest version>
flutter_datetime_picker: This will show DateTime picker.
intl: This dependency set format of choose selected date or time.
- Add the below code in your main.dart file for import dependency.
import 'package:flutter_datetime_picker/flutter_datetime_picker.dart'; import 'package:intl/intl.dart';
Now we are ready for Coding the component.
Date Picker component code.
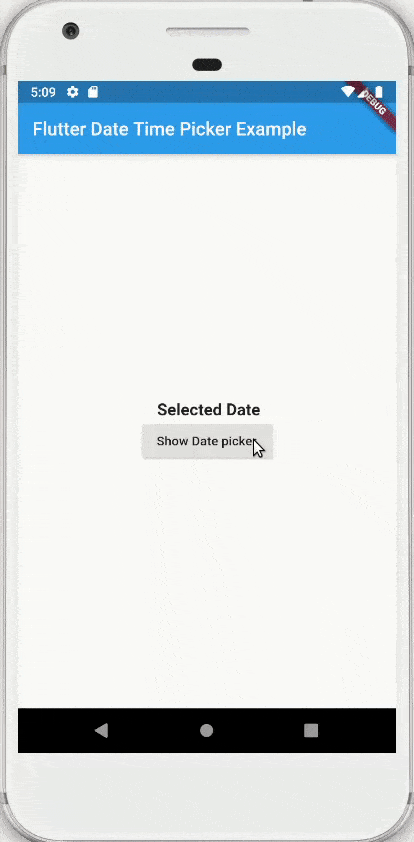
String _selectedDate;
Column( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.center, children: <Widget>[ Text(" ${_selectedDate??'Selected Date'}",style: TextStyle(fontWeight: FontWeight.bold,fontSize: 18),), RaisedButton( onPressed: () { DatePicker.showDatePicker(context, showTitleActions: true, minTime: DateTime(1980, 1, 1), maxTime: DateTime(3000, 12, 31), onConfirm: (date) {setState(() { _selectedDate=DateFormat("yyyy-MM-dd").format(date); });}, locale: LocaleType.en);},child: Text("Show Date picker"), ) ], )
Time Picker component code.
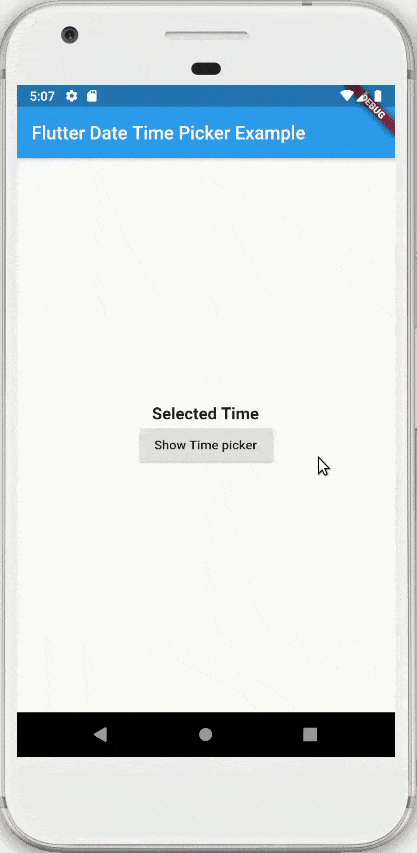
String _selectedTime;
Column( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.center, children: <Widget>[ Text("${_selectedTime??'Selected Time'}",style: TextStyle(fontWeight: FontWeight.bold,fontSize: 18),), RaisedButton( onPressed: () { DatePicker.showTimePicker(context, showTitleActions: true, onConfirm: (time) {setState(() { _selectedTime=DateFormat("HH-mm-ss").format(time); });}, locale: LocaleType.en);},child: Text("Show Time picker"), ), ], )
DateTime Picker component code.
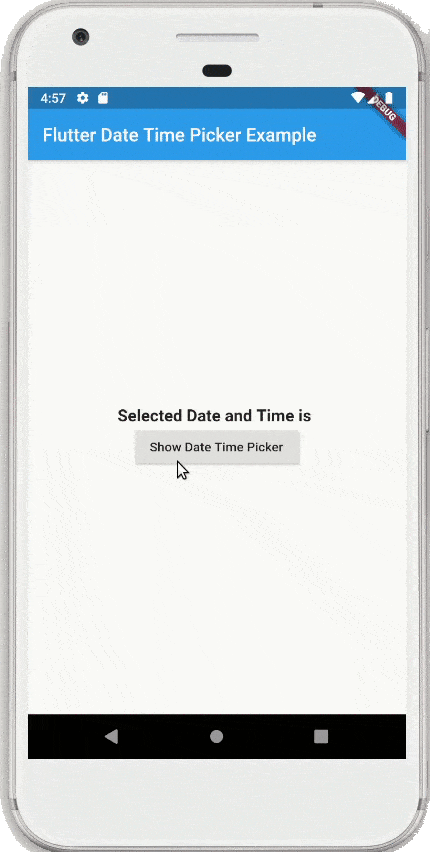
String _selectedDateTime;
Column( mainAxisAlignment: MainAxisAlignment.center, crossAxisAlignment: CrossAxisAlignment.center, children: <Widget>[ Text("${_selectedDateTime??"Selected Date and Time"}",style: TextStyle(fontWeight: FontWeight.bold,fontSize: 18),), RaisedButton( onPressed: () { DatePicker.showDateTimePicker(context, showTitleActions: true, minTime: DateTime(1980, 1, 1), maxTime: DateTime(3000, 12, 31), onConfirm: (dateTime) {setState(() { _selectedDateTime=DateFormat("yyyy-MM-dd HH-mm-ss").format(dateTime); });}, locale: LocaleType.en);},child: Text("Show Date Time Picker"), ), ], )
Language Options
In this library, you get various language options so that you can make it easy for worldwide use. For modifying the language of the component amend just update LocaleType property.
locale: LocaleType.en
You can choose date / time / date&time in below languages:
- Arabic(ar)
- Armenian(hy)
- Azerbaijan(az)
- Basque(eu)
- Bengali(bn)
- Bulgarian(bg)
- Catalan(cat)
- Chinese(zh)
- Danish(da)
- Dutch(nl)
- English(en)
- French(fr)
- German(de)
- Indonesian(id)
- Italian(it)
- Japanese(jp)
- Kazakh(kk)
- Korean(ko)
- Persian(fa)
- Polish (pl)
- Portuguese(pt)
- Russian(ru)
- Spanish(es)
- Thai(th)
- Turkish(tr)
- Vietnamese(vi)
Get complete code on GitHub.